Make Content Revealed on Hover/Focus Dismissible, Hoverable, and Persistent
Intro Text: Where additional content (like tooltips or submenus) appears on pointer hover or keyboard focus, specific conditions must be met:
- Dismissible: A way must exist to dismiss the additional content without moving pointer hover or keyboard focus (e.g., pressing Esc).
- Hoverable: The user must be able to move the pointer over the additional content without it disappearing.
- Persistent: The additional content must remain visible until the hover or focus trigger is removed, the user dismisses it, or the information is no longer valid.
Why It Matters
Users with low vision often use screen magnification, and the magnified area might obscure the trigger element when they try to view the popup content. If the popup disappears when they move the mouse (“Hoverable”), or if they can’t easily dismiss it without moving focus (“Dismissible”), it becomes unusable. Popups that disappear too quickly (“Persistent”) are also problematic. These requirements ensure users have adequate time and ability to perceive and interact with the additional content.
Fixing the Issue
Implement tooltips, popovers, and submenus carefully.
- Trigger the appearance on both hover and focus.
- Ensure the popup itself is included in the area that keeps it open when hovered (don’t trigger dismissal just because the mouse moved slightly off the original trigger onto the popup).
- Provide a clear way to dismiss, such as the Esc key or sometimes a close button (ensure the close button itself is hoverable/focusable).
- Avoid using title attributes for important information, as they often fail these criteria (especially hoverability and keyboard accessibility). Use custom tooltips built with HTML/CSS/JS and ARIA.
Good Code Example
(Conceptual – requires JavaScript) A tooltip structure designed for compliance:
-
<button id="trigger" aria-describedby="tooltip-content">Info</button> <div id="tooltip-content" role="tooltip" class="tooltip" hidden> This is the extra information. It stays visible when hovered. <button onclick="dismissTooltip()" aria-label="Close tooltip">X</button> </div> <script> const trigger = document.getElementById('trigger'); const tooltip = document.getElementById('tooltip-content'); let tooltipVisible = false; let hideTimeout; function showTooltip() { clearTimeout(hideTimeout); // Cancel any pending hide tooltip.hidden = false; tooltipVisible = true; } function scheduleHideTooltip() { // Use a small delay to allow moving pointer onto tooltip hideTimeout = setTimeout(() => { if (!tooltip.matches(':hover')) { // Hide only if not hovering tooltip dismissTooltip(); } }, 200); } function dismissTooltip() { tooltip.hidden = true; tooltipVisible = false; } // Show on focus and hover for trigger trigger.addEventListener('focus', showTooltip); trigger.addEventListener('mouseover', showTooltip); // Schedule hide on blur/mouseleave from trigger trigger.addEventListener('blur', scheduleHideTooltip); trigger.addEventListener('mouseleave', scheduleHideTooltip); // Keep open if focus/hover moves to tooltip itself tooltip.addEventListener('mouseover', showTooltip); // Cancels hide timeout tooltip.addEventListener('mouseleave', scheduleHideTooltip); tooltip.addEventListener('focusout', (event) => { // Hide if focus moves outside the tooltip+trigger component if (event.relatedTarget && !trigger.contains(event.relatedTarget) && !tooltip.contains(event.relatedTarget)) { dismissTooltip(); } }); // Dismiss on Esc key document.addEventListener('keydown', (event) => { if (event.key === 'Escape' && tooltipVisible) { dismissTooltip(); } }); </script> <style> .tooltip { position: absolute; border: 1px solid black; background-color: lightyellow; padding: 10px; width: max-content; /* Adjust as needed */ z-index: 10; } /* Add more styles for positioning */ </style>
-
This is the extra information. It stays visible when hovered.
Bad Code Example
Using a simple title attribute or a hover effect that disappears immediately:
-
<button title="This tooltip is difficult for magnification users">More Info</button> <style> .trigger .tooltip-css { display: none; position: absolute; /* ... */ } .trigger:hover .tooltip-css { display: block; } /* This tooltip disappears if the user tries to move the mouse onto it */ </style> <div class="trigger"> Hover Trigger <span class="tooltip-css">CSS Tooltip Content</span> </div>
-
Hover Trigger CSS Tooltip Content
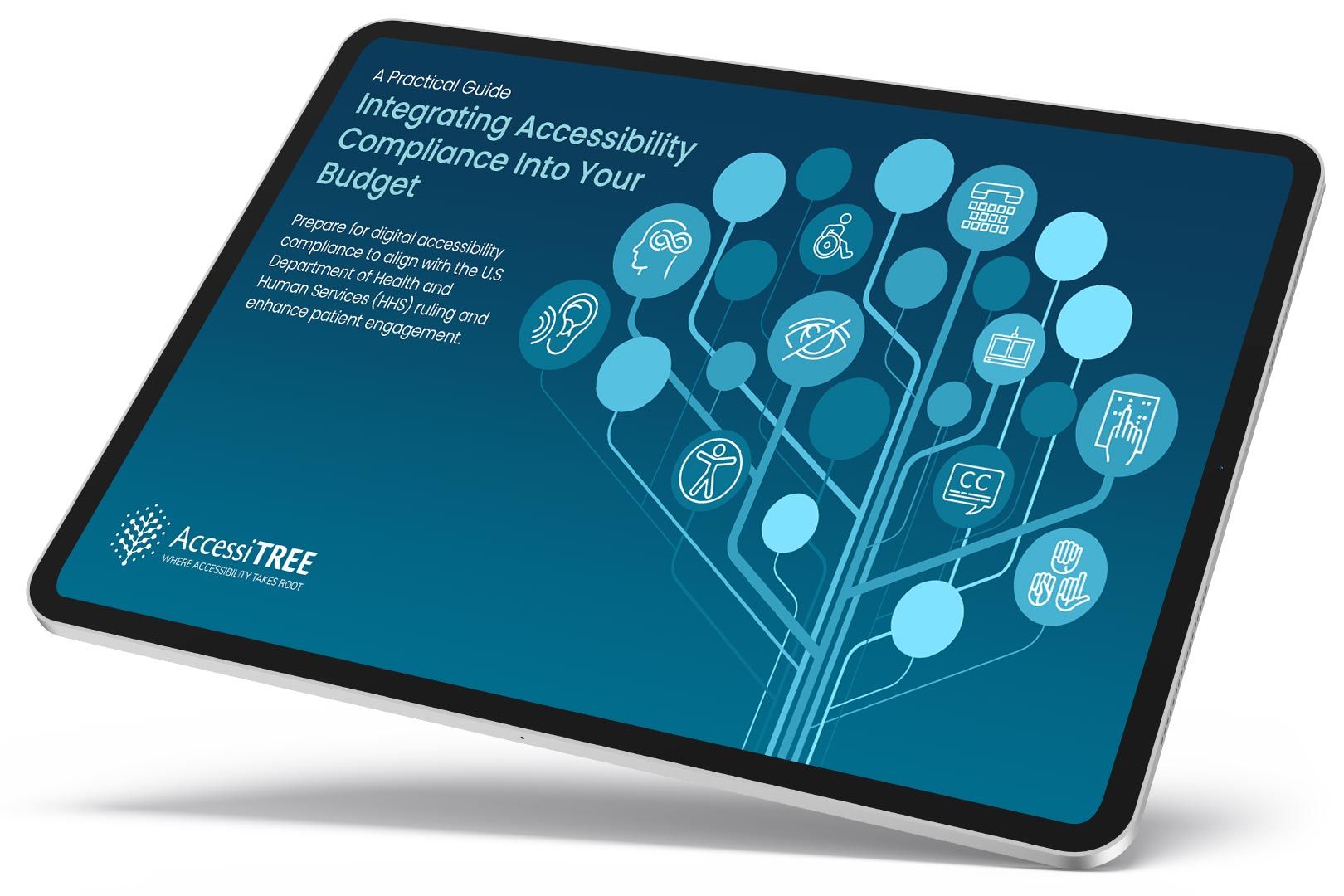
A practical guide for healthcare leaders navigating WCAG compliance.