Ensure Name, Role, and Value Are Available for All UI Components
For all user interface components (including form elements, links, and components generated by scripts), their name and role must be programmatically determinable. States, properties, and values that can be set by the user must also be programmatically settable. Notifications of changes to these items must be available to assistive technologies.
Why It Matters
Assistive technologies need to know what a component is (its role, e.g., button, checkbox, slider), what it’s called (its accessible name, e.g., “Submit”, “Subscribe to newsletter”, “Volume”), and its current state or value (e.g., checked, disabled, 50%). Without this information, users of assistive technologies cannot understand or interact with custom controls or even standard controls that haven’t been implemented correctly.
Fixing the Issue
Use standard HTML controls whenever possible, as they have built-in semantics (name, role, value, state). For custom controls (e.g., built with <div>s and JavaScript), use appropriate ARIA roles (e.g., role=”button”, role=”checkbox”), states (e.g., aria-checked, aria-disabled, aria-expanded), and properties (e.g., aria-valuenow for sliders). Ensure the control has an accessible name, typically provided by its text content, an associated <label>, aria-label, or aria-labelledby. When the state of a control changes via script, update the relevant ARIA attributes accordingly (e.g., toggle aria-checked=”true”/”false”).
Good Code Example
A custom checkbox using ARIA:
-
<span id="checkbox-label">Subscribe to newsletter</span> <div role="checkbox" tabindex="0" aria-checked="false" aria-labelledby="checkbox-label" onclick="toggleCheckbox(this)" onkeydown="handleCheckboxKeyDown(event, this)"> <span class="visual-indicator"></span> </div> <script> function toggleCheckbox(checkbox) { const currentState = checkbox.getAttribute('aria-checked') === 'true'; checkbox.setAttribute('aria-checked', !currentState); // Update visual style based on new state } function handleCheckboxKeyDown(event, checkbox) { if (event.key === ' ') { event.preventDefault(); toggleCheckbox(checkbox); } } </script> <style> /* Basic styling for the custom checkbox */ [role="checkbox"] { display: inline-block; border: 1px solid #ccc; width: 1em; height: 1em; margin-right: 5px; vertical-align: middle; cursor: pointer; } [role="checkbox"][aria-checked="true"] .visual-indicator::after { content: '✔'; } /* Example visual check */ [role="checkbox"]:focus { outline: 2px solid blue; } </style>
-
Subscribe to newsletter
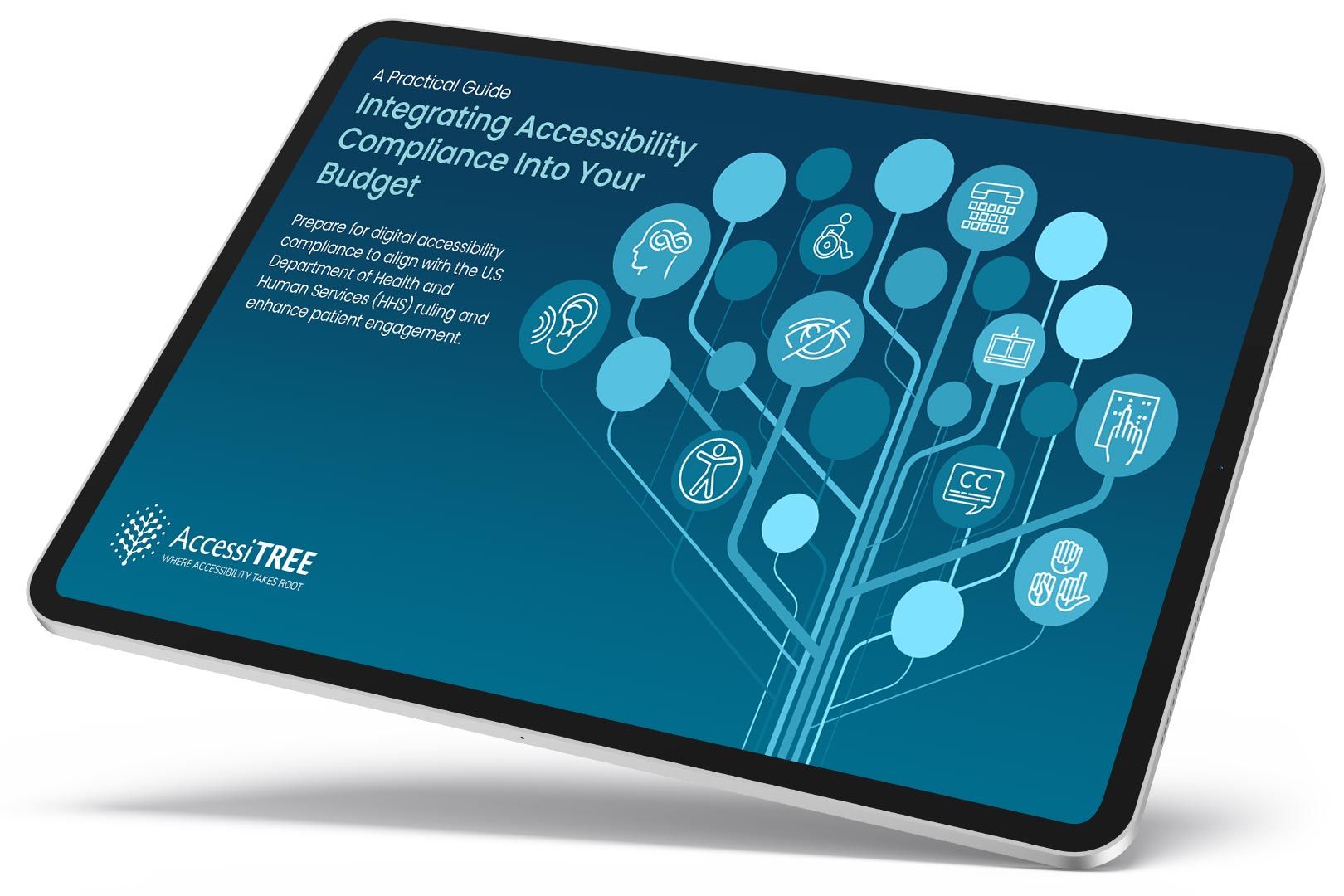
A practical guide for healthcare leaders navigating WCAG compliance.