Ensure All Functionality is Keyboard Accessible
Why It Matters
People with physical disabilities may be unable to use a mouse and rely entirely on keyboards or keyboard emulators (like switch devices). Screen reader users also navigate primarily via keyboard. If elements aren’t keyboard accessible, these users cannot interact with the website or application effectively. A visible focus indicator shows keyboard users where they are on the page. Keyboard traps prevent users from navigating away from a component.
Fixing the Issue
Use standard HTML controls like <button>, <a>, <input>, <select>, <textarea> as they are keyboard accessible by default. If creating custom interactive components (e.g., using <div> or <span> with JavaScript click handlers), ensure they are focusable (add tabindex=”0″), have appropriate ARIA roles (e.g., role=”button”), and respond to keyboard events (Enter/Space keys). Avoid removing default focus outlines unless you provide a clear, highly visible custom alternative. Test navigation thoroughly using only the Tab key (and Shift+Tab) and ensure you can activate all controls. Ensure focus can always be moved away from any element.
Good Code Example
Standard HTML elements are inherently keyboard accessible. Custom elements need extra work:
-
<button onclick="doSomething()">Click Me<a href="/about/">About Us</a><div role="button" tabindex="0" onclick="doSomething()" onkeydown="handleKeyDown(event)"> Custom Action </div><script> function handleKeyDown(event) { // Trigger action on Enter or Space key press if (event.key === 'Enter' || event.key === ' ') { event.preventDefault(); // Prevent default space bar scroll doSomething(); } } function doSomething() { console.log('Action performed!'); } </script><style> /* Ensure focus is visible (browser default is often sufficient) */ /* Or provide a custom, highly visible style */ *:focus { outline: 2px solid blue; outline-offset: 2px; } [role="button"]:focus { /* Style for custom element focus */ outline: 2px solid blue; outline-offset: 2px; } </style></button>
Bad Code Example
Using non-interactive elements for actions without making them keyboard accessible:
-
<div onclick="doSomething()">Click Here (Not Keyboard Accessible)<a href="javascript:void(0)" onclick="doSomething()">Action Link</a><style> /* Hiding focus completely - very bad practice */ *:focus { outline: none; } </style><script> function doSomething() { console.log('Action performed!'); } </script></div>
-
Click Here (Not Keyboard Accessible)Action Link
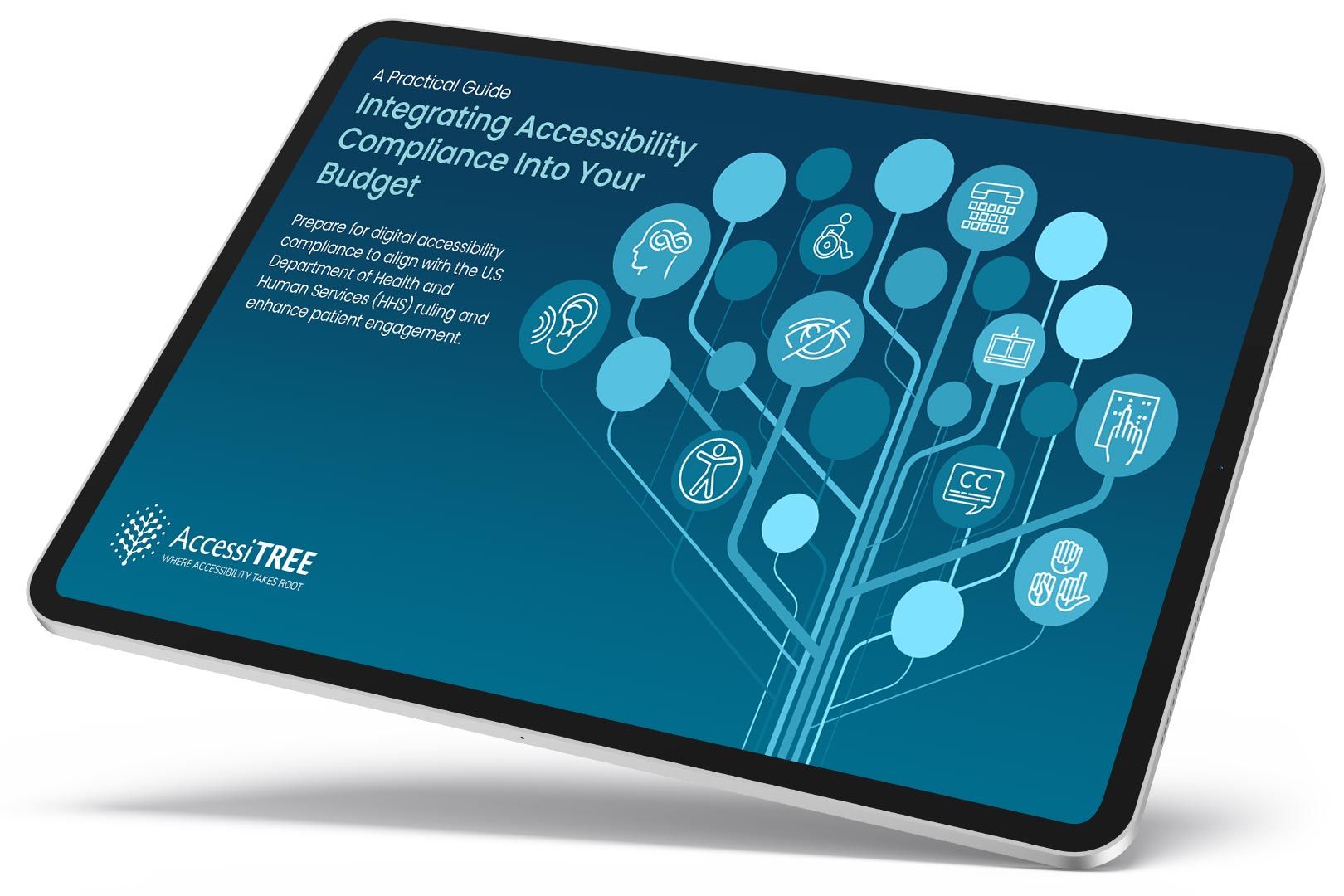