Allow Users to Turn Off, Adjust, or Extend Time Limits
For each time limit set by the content (e.g., session timeouts, timed quizzes, delays before content changes), at least one of the following must be true:
- Turn off: The user can turn the time limit off before encountering it.
- Adjust: The user can adjust the time limit before encountering it over a wide range (at least ten times the default setting).
- Extend: The user is warned before time expires and given at least 20 seconds to extend the time limit with a simple action (like pressing the space bar), and the user can extend the limit at least ten times.
- (Exceptions exist for real-time events or where the time limit is essential).
Why It Matters
Users with various disabilities may require more time to read content, understand instructions, or perform actions. Automatically timing out sessions or advancing content can prevent these users from completing tasks successfully. Providing control over time limits ensures users have sufficient time.
Fixing the Issue
Avoid unnecessary time limits. If a time limit is necessary (e.g., for security reasons like session timeouts), implement a mechanism to warn the user before the limit expires (e.g., a modal dialog). Provide simple controls within that warning to extend the session easily (e.g., an “Extend Session” button). Ensure the warning gives at least 20 seconds notice and allows multiple extensions. For timed activities like tests, consider allowing users to request extended time or disable the timer beforehand if possible.
Good Code Example
(Conceptual – requires JavaScript) Warning before session timeout with an extension option:
-
<div id="timeout-warning" role="alertdialog" aria-labelledby="timeout-heading" aria-describedby="timeout-desc" hidden> <h2 id="timeout-heading">Session Timeout Warning</h2> <p id="timeout-desc">Your session is about to expire due to inactivity. You will be logged out in <span id="time-remaining">60</span> seconds.</p> <button onclick="extendSession()">Extend Session</button> <button onclick="logoutNow()">Log Out Now</button> </div> <script> let sessionTimeout = 15 * 60 * 1000; // 15 minutes let warningTime = 60 * 1000; // 1 minute warning let inactivityTimer; let warningTimer; let countdownTimer; let timeRemaining = warningTime / 1000; function showWarning() { const dialog = document.getElementById('timeout-warning'); timeRemaining = warningTime / 1000; document.getElementById('time-remaining').textContent = timeRemaining; dialog.hidden = false; // Start countdown countdownTimer = setInterval(() => { timeRemaining--; document.getElementById('time-remaining').textContent = timeRemaining; if (timeRemaining <= 0) { logoutNow(); } }, 1000); // Focus the dialog or extend button for keyboard users dialog.querySelector('button').focus(); } function resetTimer() { clearTimeout(inactivityTimer); clearTimeout(warningTimer); clearInterval(countdownTimer); document.getElementById('timeout-warning').hidden = true; // Warn 1 minute before actual timeout warningTimer = setTimeout(showWarning, sessionTimeout - warningTime); // Actual logout timer inactivityTimer = setTimeout(logoutNow, sessionTimeout); } function extendSession() { // Make a request to server to extend session if needed console.log("Session extended"); resetTimer(); // Reset timers on client side } function logoutNow() { console.log("Logging out"); // Redirect to logout page // window.location.href = '/logout'; clearInterval(countdownTimer); } // Reset timer on user activity window.onload = resetTimer; document.onmousemove = resetTimer; document.onkeypress = resetTimer; document.onclick = resetTimer; document.onscroll = resetTimer; document.ontouchstart = resetTimer; </script>
Bad Code Example
A system that logs users out after a fixed time with no warning or option to extend:
-
// Server-side or client-side logic that simply redirects after a fixed time // without any user warning or control. const SESSION_DURATION = 15 * 60 * 1000; // 15 minutes setTimeout(() => { // Force logout without warning window.location.href = '/logout?reason=timeout'; }, SESSION_DURATION); // User is abruptly logged out, potentially losing work.
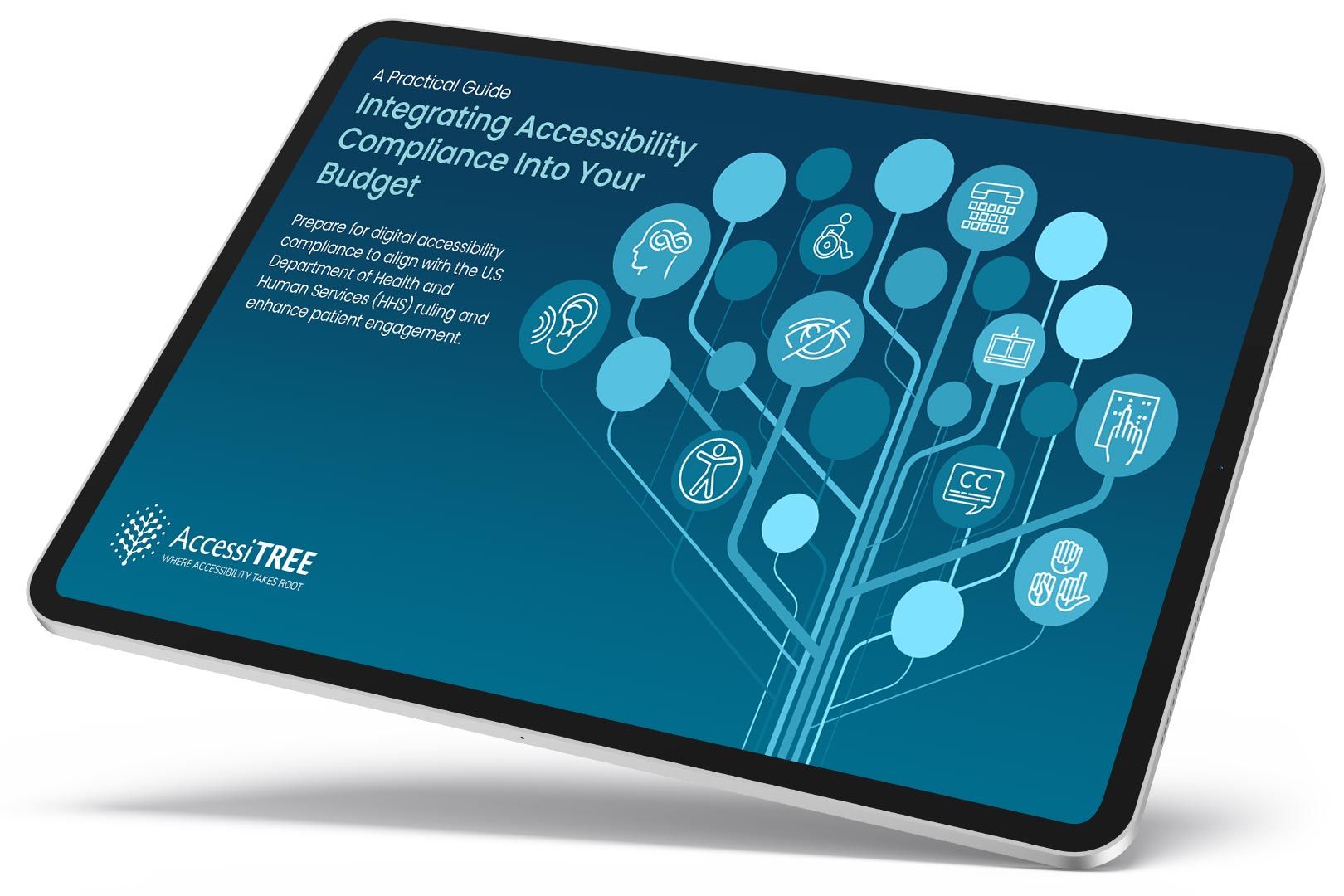
A practical guide for healthcare leaders navigating WCAG compliance.