Allow Users to Cancel Actions Triggered by Pointer Down-Events
For functionality that can be operated using a single pointer (like a mouse click or screen tap), at least one of the following must be true for the down-event (mouse button press, finger touch start):
- No down-event: The function is not executed on the down-event. (Activation happens on the up-event, like a standard click).
- Abort or Undo: Completion of the function is on the up-event, and a mechanism is available to abort the function before completion (e.g., by dragging the pointer away before releasing) or to undo the function after completion.
- Up-event reverses: The up-event reverses any outcome of the down-event.
- Essential: Completing the function on the down-event is essential (e.g., simulating a keyboard press in an on-screen keyboard).
Why It Matters
Users with motor impairments may accidentally press the mouse button or touch the screen. If the action triggers immediately on the down-event, they have no chance to correct the error before the action completes. Standard click/tap behavior triggers on the up-event, allowing users to move their finger/pointer away before releasing to cancel the action. This success criterion ensures users have a way to prevent accidental activation.
Fixing the Issue
Use standard click/tap events (onclick in JS, standard HTML links/buttons) which trigger on the up-event by default. Avoid using onmousedown or ontouchstart to trigger the primary action of a control. If you must use a down-event (e.g., for specific drag interactions), ensure the user can cancel by moving away before the up-event, or provide an undo mechanism.
Good Code Example
Using standard click event which fires on up-event:
-
<button id="action-button">Perform Action</button> <script> const button = document.getElementById('action-button'); button.addEventListener('click', function() { // This code runs on the 'up-event' (standard click) console.log('Action performed!'); // performAction(); }); // If user presses mouse down, drags away, then releases, // the 'click' event does not fire. This allows cancellation. </script>
-
Bad Code Example
Triggering an action immediately on mousedown:
-
<button id="bad-button">Perform Action Immediately</button> <script> const badButton = document.getElementById('bad-button'); // Bad: Action triggers as soon as the mouse button is pressed down. badButton.addEventListener('mousedown', function() { console.log('Action performed on mousedown! No chance to cancel by dragging away.'); // performActionImmediately(); // This fails WCAG 2.5.2 unless the action is essential or undoable/abortable. }); </script>
-
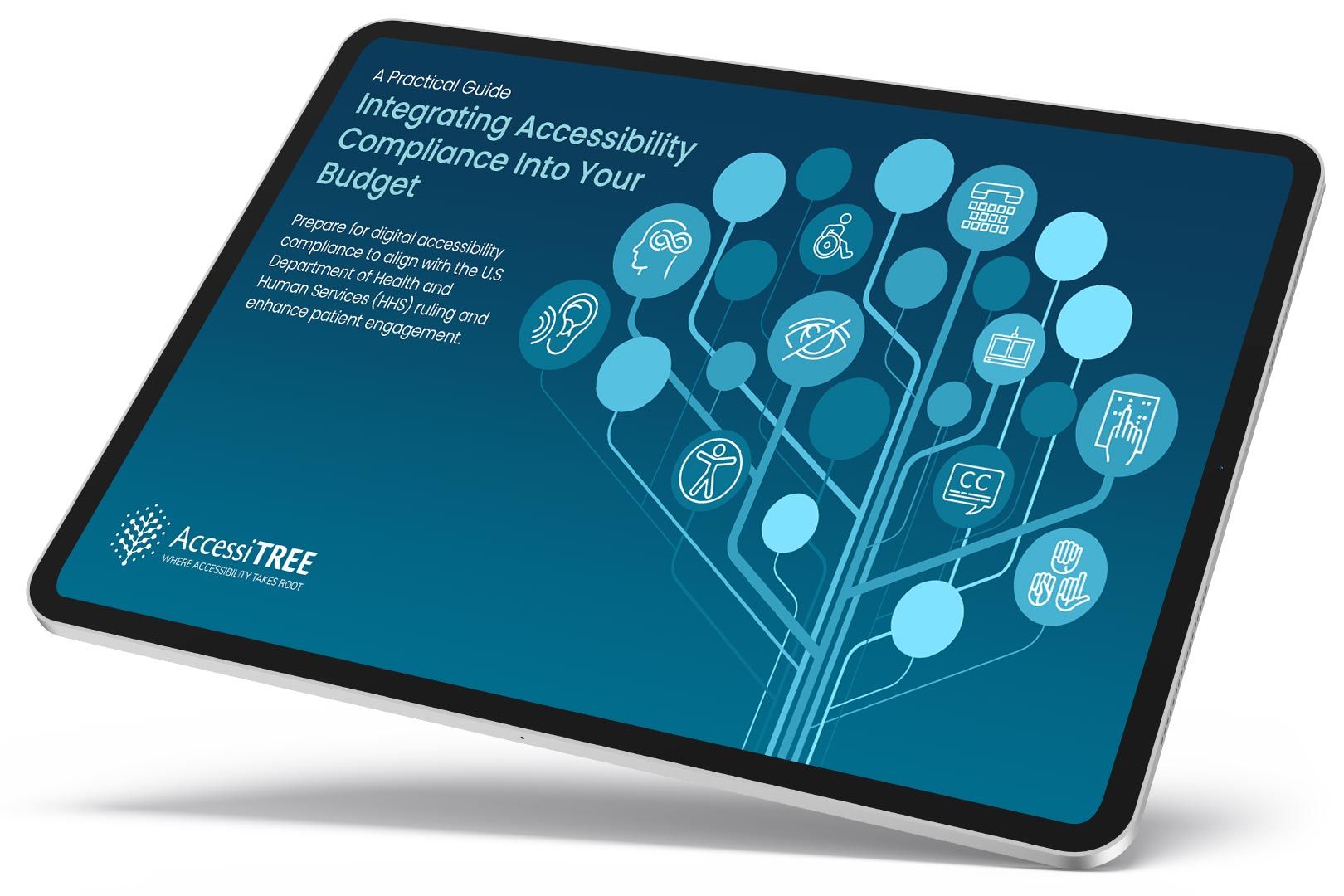
A practical guide for healthcare leaders navigating WCAG compliance.